What Are the Best Coding Practices
In the world of software development, coding practices play a crucial role in creating efficient, maintainable, and scalable applications. Adhering to best coding practices not only improves the quality of your code but also facilitates collaboration among team members and enhances the overall development process. Whether you’re a seasoned developer or just starting out, understanding and implementing best coding practices is essential for producing high-quality software.
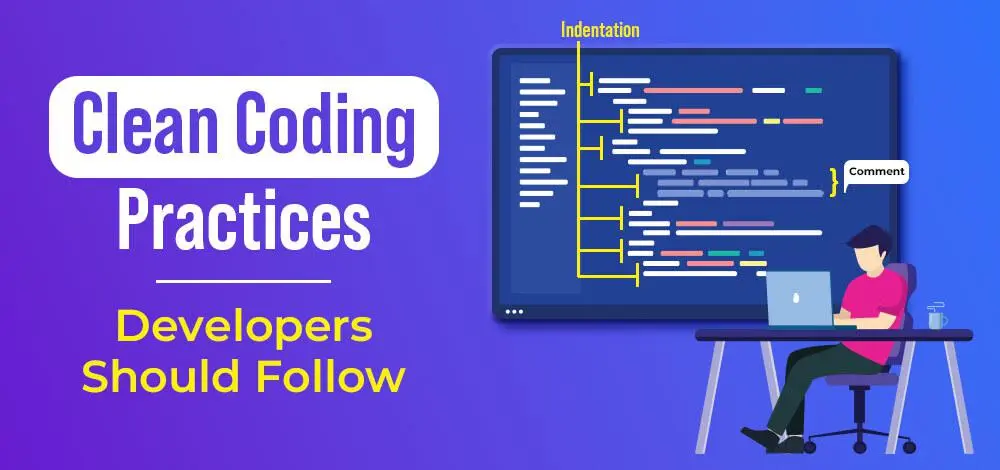
This guide will explore the best coding practices that every developer should follow to write clean, efficient, and maintainable code.
Writing Clean and Readable Code
Use Meaningful Names
One of the foundational coding practices is using meaningful and descriptive names for variables, functions, classes, and other entities in your code. Names should clearly convey the purpose of the element they represent, making the code easier to understand for anyone who reads it.
- Variables: Use descriptive names that indicate the type of data stored. For example,
userAge
is more meaningful thanua
. - Functions: Function names should clearly describe the action they perform. For instance,
calculateTotalPrice()
is better thancalcTP()
. - Classes: Class names should represent the entities they model, such as
Customer
,Order
, orInvoice
.
Follow Consistent Naming Conventions
Consistency in naming conventions is key to writing maintainable code. Whether you use camelCase, snake_case, or PascalCase, stick to one convention throughout your project. This consistency makes your codebase easier to navigate and reduces confusion.
- CamelCase: Commonly used for variables and function names (e.g.,
getUserInfo
). - PascalCase: Typically used for class names (e.g.,
OrderDetail
). - snake_case: Often used for file names and constants (e.g.,
MAX_CONNECTIONS
).
Comment Your Code Wisely
Comments are a valuable tool for explaining complex logic or providing context within your code. However, they should be used sparingly and effectively. The goal is to make your code self-explanatory, with comments providing additional clarity when necessary.
- Avoid Redundant Comments: Comments should not restate what the code is doing. Instead, they should explain the why behind the code.
- Use Comments for Complex Logic: If a particular section of code is complex or not immediately intuitive, add a comment to clarify its purpose.
- Document Functions and Classes: Include brief descriptions of what a function or class does, its parameters, and its return values.
Structuring Your Code Efficiently
Keep Functions Small and Focused
Functions should be small and focused on a single task. This practice, often referred to as the Single Responsibility Principle (SRP), makes your code easier to test, debug, and maintain.
- Avoid Long Functions: Break down long functions into smaller, more manageable functions. This not only improves readability but also promotes code reuse.
- Single Responsibility: Each function should have one clear purpose. For example, a function that handles user authentication should not also be responsible for logging user activities.
Organize Code into Modules
Modularizing your code by organizing it into smaller, self-contained modules or files is a best practice that enhances maintainability and scalability. Each module should encapsulate a specific functionality, making it easier to manage and test.
- Separate Concerns: Group related functions and classes together in separate modules. For example, you could have separate modules for user authentication, database interactions, and UI components.
- Reusability: Modular code is easier to reuse in different parts of your application or even in different projects.
Adopt DRY (Don’t Repeat Yourself) Principle
The DRY principle emphasizes reducing code duplication by abstracting repetitive code into reusable functions or classes. By following this principle, you can minimize errors and make your codebase more efficient.
- Identify Repetitive Code: Look for patterns of repetition in your code and refactor them into reusable functions or classes.
- Use Inheritance and Interfaces: In object-oriented programming, use inheritance and interfaces to avoid code duplication and promote code reuse.
Ensuring Code Quality and Performance
Write Unit Tests
Unit testing is a best practice that involves writing tests for individual units of code, such as functions or methods. Unit tests help ensure that your code works as expected and can catch bugs early in the development process.
- Test-Driven Development (TDD): Consider adopting TDD, where you write tests before writing the actual code. This approach ensures that your code is always testable and meets the requirements.
- Automate Testing: Use testing frameworks and tools to automate your unit tests, making it easier to run them frequently and catch issues early.
Optimize Code for Performance
Performance optimization is crucial for creating responsive and efficient applications. Write code that is not only functional but also optimized for speed and resource usage.
- Avoid Premature Optimization: Focus on writing clean and maintainable code first, and optimize for performance only when necessary.
- Use Efficient Algorithms: Choose the most appropriate algorithms and data structures for your use case. For example, prefer using a hash map over a list for quick lookups.
- Minimize Memory Usage: Be mindful of memory usage, especially in environments with limited resources, such as mobile devices or embedded systems.
Code Reviews
Code reviews are a critical practice for maintaining code quality. They involve having peers review your code before it is merged into the main codebase, providing an opportunity to catch bugs, improve code readability, and share knowledge.
- Collaborative Reviews: Engage in collaborative code reviews where both the author and reviewers can discuss and suggest improvements.
- Check for Best Practices: During code reviews, ensure that best coding practices are followed, such as adhering to naming conventions, writing tests, and avoiding code duplication.
Maintaining Code with Version Control
Use Version Control Systems
Version control systems (VCS) like Git are essential tools for managing changes to your codebase. They allow you to track changes, collaborate with others, and revert to previous versions of your code if necessary.
- Commit Regularly: Make frequent commits with clear, descriptive messages. This practice helps you keep track of changes and makes it easier to identify issues.
- Branching and Merging: Use branching to work on new features or fixes without affecting the main codebase. Once the changes are tested and reviewed, merge them back into the main branch.
Maintain a Clean Git History
A clean and organized Git history is beneficial for understanding the evolution of your project and for debugging. Avoid cluttering the history with unnecessary commits or merge conflicts.
- Squash Commits: When merging feature branches, consider squashing commits to combine them into a single commit with a clear summary of the changes.
- Rebase vs. Merge: Understand when to use rebasing and when to merge branches. Rebasing can create a linear history, while merging preserves the context of individual commits.
Documenting Your Code and Processes
Write Clear Documentation
Comprehensive documentation is a cornerstone of good coding practices. It helps other developers (and future you) understand the codebase, set up the environment, and use the software effectively.
- Inline Documentation: Use inline comments and docstrings to document the purpose and usage of functions, classes, and modules.
- External Documentation: Create external documentation that includes an overview of the project, installation instructions, API references, and usage examples.
Maintain an Updated README File
The README file is often the first point of contact for new developers or users of your project. Keep it up to date with essential information, including project description, setup instructions, and contribution guidelines.
- Project Overview: Provide a brief summary of what the project does and its main features.
- Getting Started: Include clear instructions on how to set up the development environment and run the project.
- Contribution Guidelines: Outline how others can contribute to the project, including coding standards, pull request procedures, and code review policies.
Continuous Learning and Improvement
Stay Updated with Industry Trends
The field of software development is constantly evolving, with new tools, languages, and practices emerging regularly. Stay informed about the latest trends and best practices to keep your skills and knowledge up to date.
- Follow Blogs and Forums: Read industry blogs, participate in forums, and follow influential developers on social media to stay informed about the latest developments.
- Attend Workshops and Conferences: Participate in workshops, webinars, and conferences to learn from experts and network with other professionals.
Refactor and Improve Your Code
Refactoring is the process of improving the structure and readability of your code without changing its functionality. Regularly revisit and refactor your code to improve its quality, address technical debt, and keep it aligned with best practices.
- Identify Code Smells: Look for signs of poorly written code, such as long functions, duplicated code, or complex logic, and refactor them to improve clarity and maintainability.
- Iterative Improvements: Adopt an iterative approach to refactoring, making small, incremental improvements over time rather than attempting large-scale changes all at once.
Conclusion
Adopting best coding practices is essential for writing high-quality, maintainable, and efficient code. By following the guidelines outlined in this article—such as writing clean and readable code, structuring it efficiently, ensuring code quality, and maintaining thorough documentation—you can enhance your development process and create software that is robust and scalable. Continuous learning and improvement are key to staying ahead in the ever-evolving field of software development. By embracing these best practices, you’ll not only improve your own coding skills but also contribute to the